Adding Twitter Card and Facebook Sharing Support to Tumblelog
August 11, 2019
In the afternoon I added a new template variable to tumblelog
, both
the Perl and the Python version. Thanks to this additional variable,
page-url
, it's easy to add support for Twitter card and Facebook
sharing to a static blog generated with
tumblelog
.
Modifying the Blog Template
After you have done a git pull
add the following lines before the
line containing </head>
in the template you use for your blog:
<meta property="og:url" content="[% page-url %]">
<meta property="og:type" content="article">
<meta property="og:title" content="[% title %]">
<meta property="og:image"
content="http://example.com/images/site-logo.png">
<meta property="og:description" content="Link to: [% page-url %]">
<meta name="twitter:card" content="summary">
<meta name="twitter:image:alt" content="SOME SITE logo">
You have to modify the following for your own blog:
- provide a full path to a logo image for the
og:image
property. - provide a text describing the logo for the
twitter:image:alt
property.
For my tumblelog, Plurrrr, I created a simple
512x512 pixels logo showing an orange P. Because I use the summary
Twitter card this logo is shown to the left of the title and description:
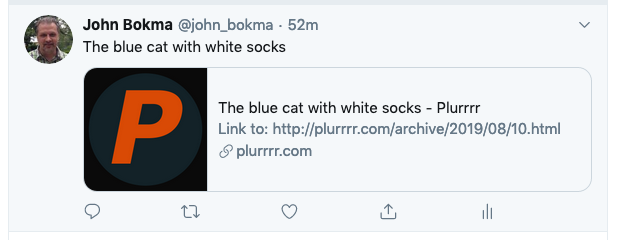
Sadly, a rectangular cut-out is shown on Facebook, but since I made
this mostly for Twitter I don't care much. If you prefer a rectangular
logo set the content
attribute of twitter:card
to
summary_large_image
. For more information, please read Twitter Card
and Facebook
Sharing.
Generating Tweets
I use a Perl program called tweetfile.pl to post a tweet at random chosen from a text file in fortune cookie format.
A simple Perl program, given below which I named tweets.pl
, can be
used to create such a file from the Markdown file used as input for
tumblelog
.
Edit: I have updated the program to handle UTF-8 correctly.
#!/usr/bin/perl
my $filename = shift;
defined $filename or die "Usage: tweets.pl FILENAME.MD\n";
binmode STDOUT, ':encoding(UTF-8)';
open my $fh, '<:encoding(UTF-8)', $filename
or die "Can't open '$filename' for reading: $!";
while ( my $line = <$fh> ) {
$line =~ /^(\d{4})-(\d{2})-(\d{2})\s+(.+)/ or next;
print <<"END_TWEET"
$4
\x{1f449} Please retweet if you like my #blog Thanks! \x{1f44d}
https://plurrrr.com/archive/$1/$2/$3.html
%
END_TWEET
}
You call it as follows:
perl tweets.pl site.md > site-tweets.txt
The program iterates over all lines in the given Markdown file and if a match is found of a date followed by a title a tweet is printed to the standard output which is redirected to a text file.
Don't forget to replace the URL in this Perl program with the one to your blog.
One caveat of this program though, if you have a blog post which has a line starting with a date in year-month-day format, for example:
2019-08-11 This is an example
this will also be seen as a blog post; a false positive. Writing a
program that avoids this special case is left as an exercise to the
reader; you could use code from tumblelog
, either the Perl or Python
version, as a starting point.
Automating
As I use a Makefile to generate my
tumblelog it's easy to call the
aforementioned tweets.pl
Perl program as part of the make process:
TUMBLELOG = ../../projects/tumblelog
TWITTERTOOLS=../../projects/twitter-tools
PERL = perl
SCSS = soothe.scss
SASS = sass --sourcemap=none -t compressed
CSS = soothe.css
TEMPLATE = plurrrr.html
AUTHOR = 'John Bokma'
BLOG_URL = http://plurrrr.com/
NAME = 'Plurrrr'
all: local tweets
rsync -avh --delete -c htdocs/ ti:sites/plurrrr.com/public/
tweets:
@perl tweets.pl plurrrr.md > $(TWITTERTOOLS)/plurrrr-tweets.txt
local:
@echo "Building latest version of blog using Perl"
@$(SASS) $(TUMBLELOG)/styles/$(SCSS) htdocs/$(CSS)
@$(PERL) $(TUMBLELOG)/tumblelog.pl --output-dir htdocs \
--template $(TEMPLATE) --css $(CSS) \
--author $(AUTHOR) --blog-url $(BLOG_URL) \
--name $(NAME) --quiet plurrrr.md
Note that I added a new rule; tweets
and added this as a dependency
to the all
rule. Don't forget the latter.
Now, if you run just make
the tweets
target should run and create
the text file with tweets.
You can use a cron
job to tweet automatically, daily, as explained in
Running a Perl program via
cron.